import ‘package:path/path.dart’;
import ‘package:sqflite/sqflite.dart’;
import ‘../models/product.dart’;
class DbHelper{
late
Database _db;
Future<Database> get db async{
if(_db==null){
_db = await initializeDb();
}
return _db;
}
Future<Database> initializeDb() async {
String dbPath = join(await getDatabasesPath(),“etrade.db”);
var eTradeDb = await openDatabase(dbPath,version: 1, onCreate: createDb);
return eTradeDb;
}
void createDb(Database db, int version) async {
await db.execute(“Create table products(İd integer primary key, name text, description text, unitPrice integer)”);
}
Future<List<Product>> getProducts() async {
Database db = await this.db;
var result = await db.query(“products”);
return List.generate(result.length, (i) {
return Product.fromObject(result);
});
}
Future<int> insert(Product product) async {
Database db = await this.db;
var result = await db.insert(“products”,product.toMap());
}
Future<int> delete(int id) async {
Database db = await this.db;
var result = await db.rawDelete(“delete form products where id= $id”);
return result;
}
Future<int> update(Product product) async {
Database db = await this.db;
var result = await db.update(“products”, product.toMap(), where: “id=?”,whereArgs: [product.id]);
return result;
}
}
class Product{
late int id;
late String name;
late String description;
late double unitPrice;
Product(this.name, this.description, this.unitPrice);
Product.withId(this.id,this.name, this.description, this.unitPrice);
Map<String,dynamic> toMap(){
var map = Map<String,dynamic>();
map["name"]=name;
map["description"]=description;
map["unitPrice"]=unitPrice;
if(id!=null){
map["id"]=id;
}
}
Product.fromObject(dynamic o){
this.id = int.tryParse(o[“İd”]);
this.name = o[“name”];
this.description = o[“description”];
this.unitPrice = double.tryParse(o[“unitPrice”]);
}
}
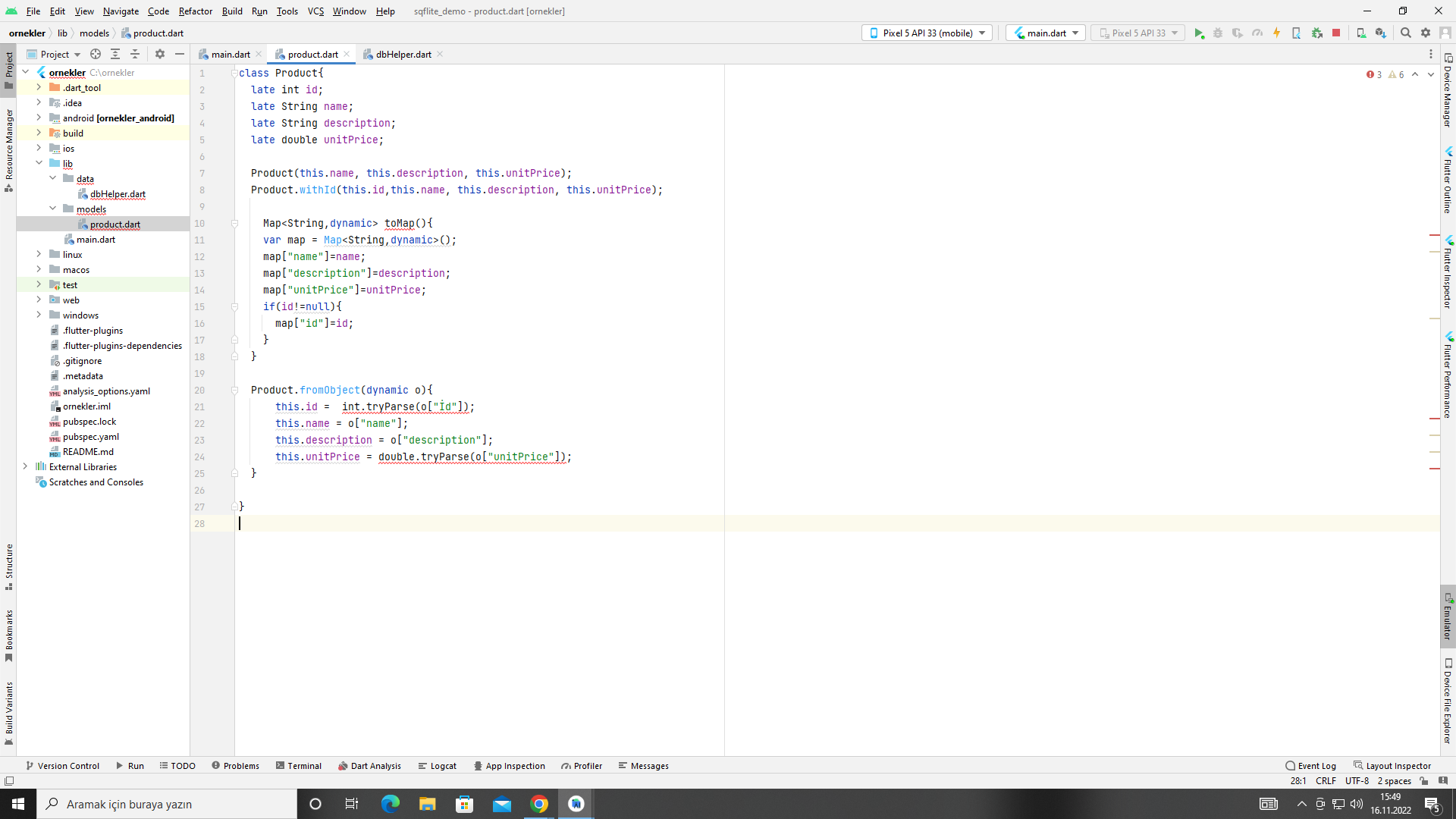
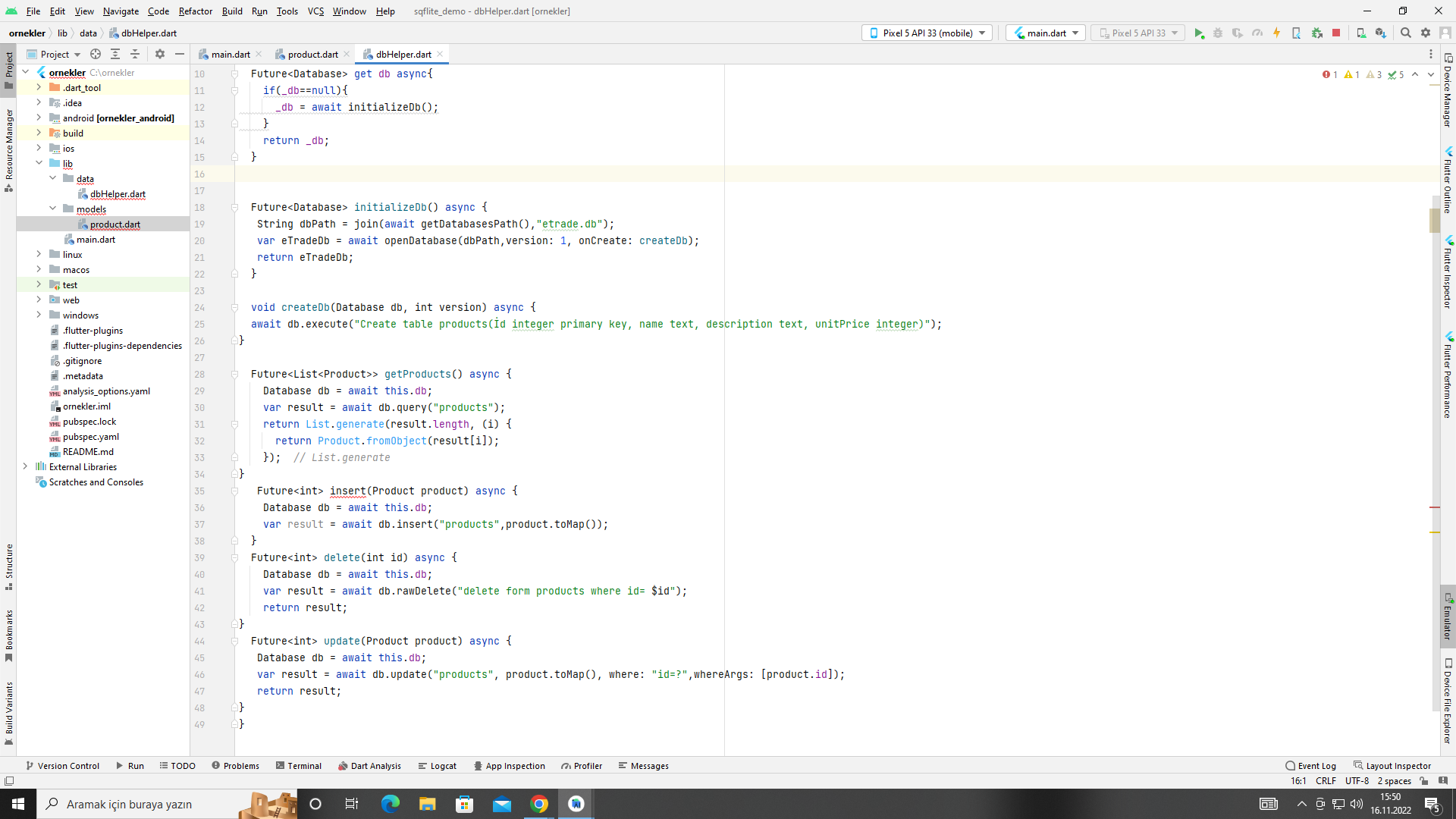
RESİM LİNKLERİ : 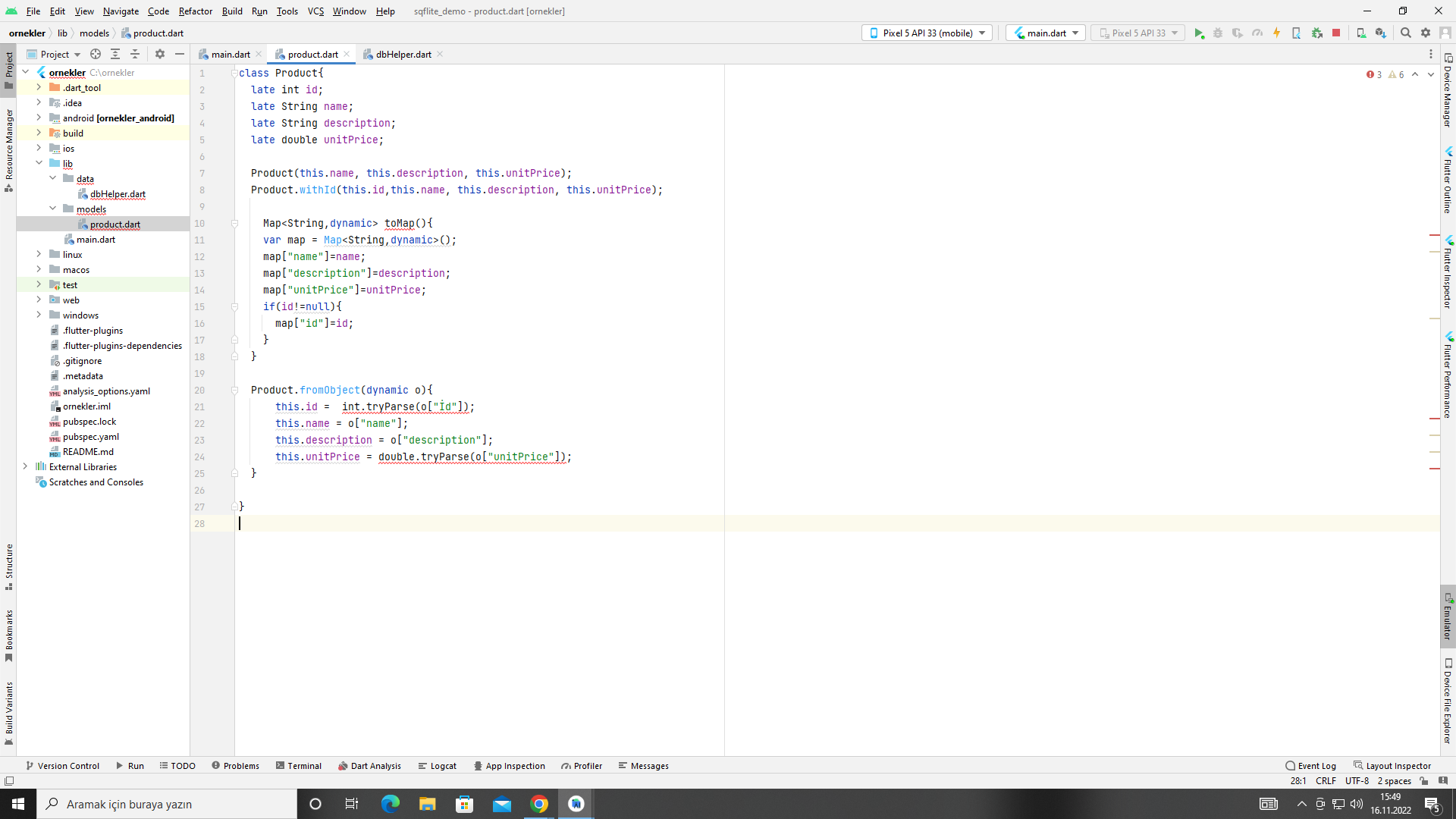
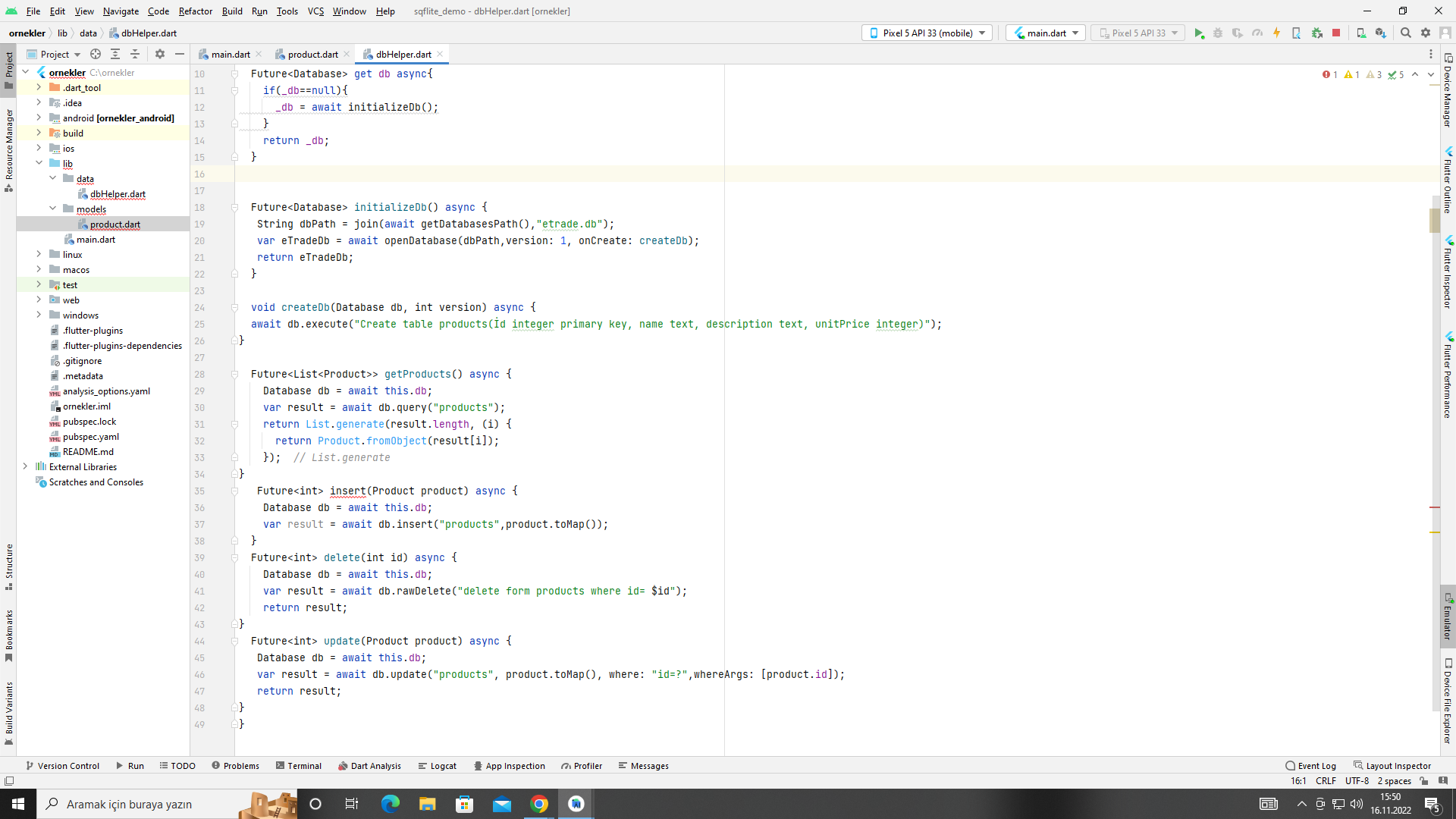